Circular Single Linked List
• In
circular, last node contains a pointer to the first node
• We
can have a circular singly linked list as well as a circular doubly linked
list.
• There
is no storing of NULL values in the list

Advantages of Circular Linked Lists:
1) Any node can be a starting point.
2) Useful for implementation of queue.
3) Circular lists are useful in applications to repeatedly go around the list.
4) Circular Doubly Linked Lists are used for implementation of advanced data structures like Fibonacci Heap.
Doubly Linked List
• Double/Doubly
linked list (DLL) or two-way linked list is a linked
list data structure with two link, one that contain reference to the next data
and one that contain reference to the previous data.
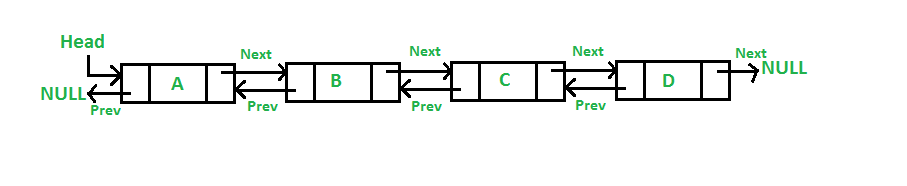
};
Advantages over singly linked list:
1) A DLL can be traversed in both forward and backward direction.
2) The delete operation in DLL is more efficient if pointer to the node to be deleted is given.
3) We can quickly insert a new node before a given node.
Disadvantages over singly linked list
1) Every node of DLL Require extra space for an previous pointer.
1) Every node of DLL Require extra space for an previous pointer.
2) All operations require an extra pointer previous to be maintained.
Doubly Linked List: Insertion
A node can be added in four ways:1) At the front of the DLL.
2) After a given node.
3) At the end of the DLL.
4) Before a given node.
Doubly Linked List: Deletion
There are 4 conditions we should
pay attention when deleting:
1) The node to be deleted is the only
node in linked list.
2) The node to be deleted is head.
3) The node to be deleted is tail.
4) The node to be deleted is not head
or tail.
Circular Doubly Linked List
Circular Doubly Linked List has properties of both doubly linked list and circular linked list in
which two consecutive elements are linked or connected by previous and next pointer and
the last node points to first node by next pointer and also the first node points to last node
by previous pointer.
That is my summary for Data Structure Session 3 on 24th February 2020, Thank you
Source:
Source:
Tidak ada komentar:
Posting Komentar